Stacks 101: An Introduction to the Abstract Data Type
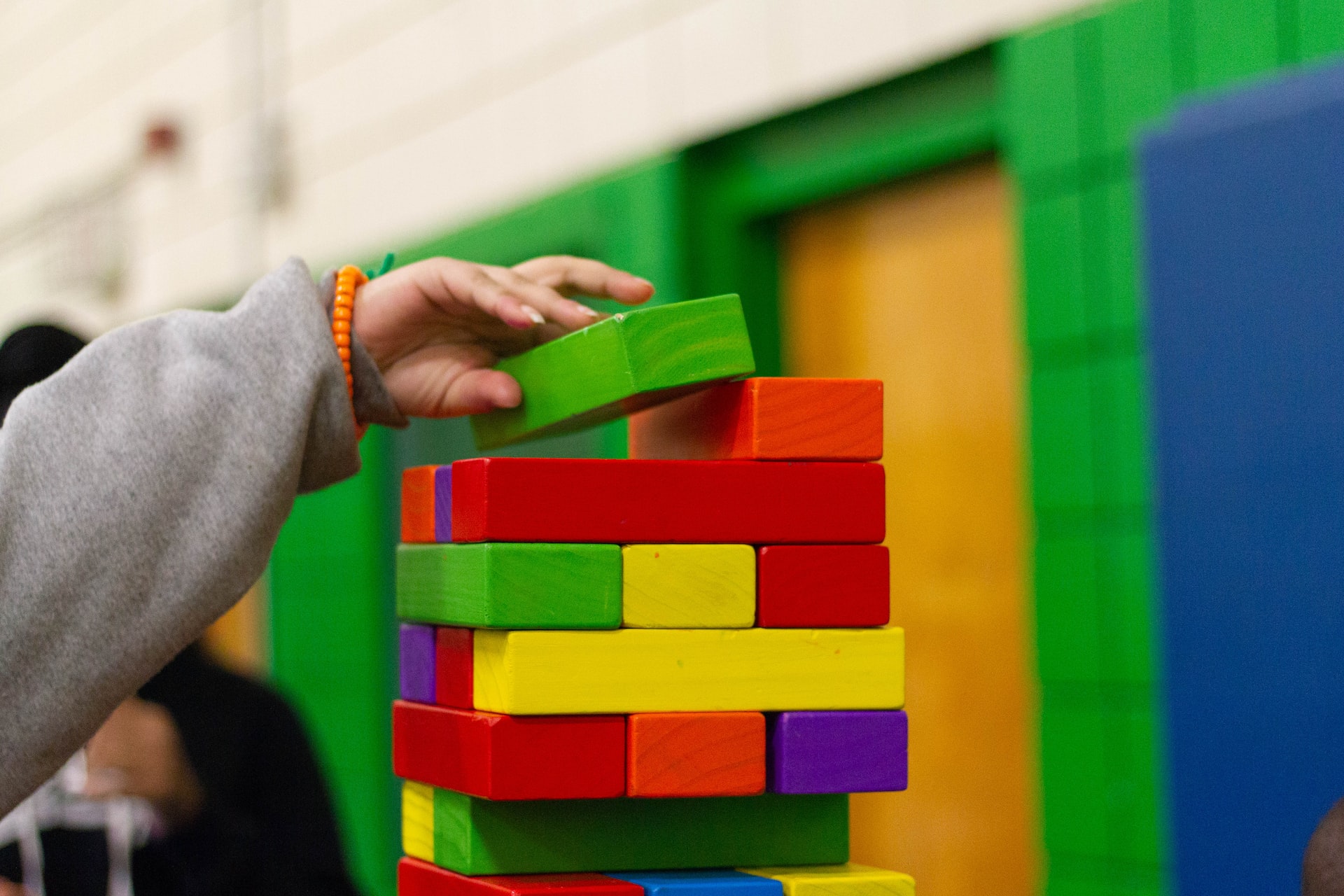
A stack is a fundamental data structure in computer science that is used to store and organize data in a specific order. It is an abstract data type, meaning that it is a logical representation of a data structure and not a specific implementation.
In this article, we will provide an introduction to stacks, including the basic principles of how they work and how they are used in computer science.
Basic Principles of Stacks
A stack is a Last-In-First-Out (LIFO) data structure, meaning that the last element added to the stack is the first element to be removed. This is in contrast to a First-In-First-Out (FIFO) data structure, like a queue, where the first element added is the first element to be removed.
The basic operations that can be performed on a stack are push, pop, and peek. The push operation adds an element to the top of the stack, the pop operation removes the top element from the stack, and the peek operation returns the value of the top element without removing it.
Implementation of Algorithms
One of the main uses of stacks in computer science is in the implementation of algorithms, particularly recursive algorithms. A recursive algorithm is one that calls itself in order to solve a problem, and it requires the use of a stack to keep track of the function calls.
The stack stores the current state of the function, including the parameters and the return address, so that the function can return to its previous state once it has been completed. This is known as the stack frame, and it is important in the context of memory management.
Expression Parsing
Another application of stacks is in the parsing of expressions, such as mathematical expressions or programming expressions. A stack can be used to evaluate expressions by converting them from infix notation to postfix notation.
Infix notation is the standard way of writing expressions, where the operator is placed between the operands, for example, 2+3. On the other hand, postfix notation is where the operator comes after the operands, for example, 2 3 +, in this notation it’s easier to evaluate the expression by using a stack.
Balancing Symbols
Stacks can also be used to check for balanced symbols, such as parentheses, brackets, or curly braces. This is done by using a stack to keep track of the opening symbols as they are encountered in the input, and then checking that they are matched with the corresponding closing symbols in the correct order.
Memory Management
In addition to their use in algorithms and expression parsing, stacks are also used in computer systems for memory management. The stack is used to store the current state of a program, including the values of variables and the location of the instruction pointer.
When a function is called, a new stack frame is created to store the state of the function, and when the function returns, the stack frame is removed, freeing up memory for other uses.
Conclusion
In conclusion, stacks are fundamental data structures in computer science that are used to store and organize data in a specific order. They are a Last-In-First-Out (LIFO) data structure, and the basic operations that can be performed on a stack are push, pop, and peek.
Stacks have many applications in computer science, including the implementation of algorithms, expression parsing, and memory management. Understanding stacks and how to use them effectively is an essential skill for computer scientists and software developers.