Creating Animated GIFs in Google Colab Using MoviePy
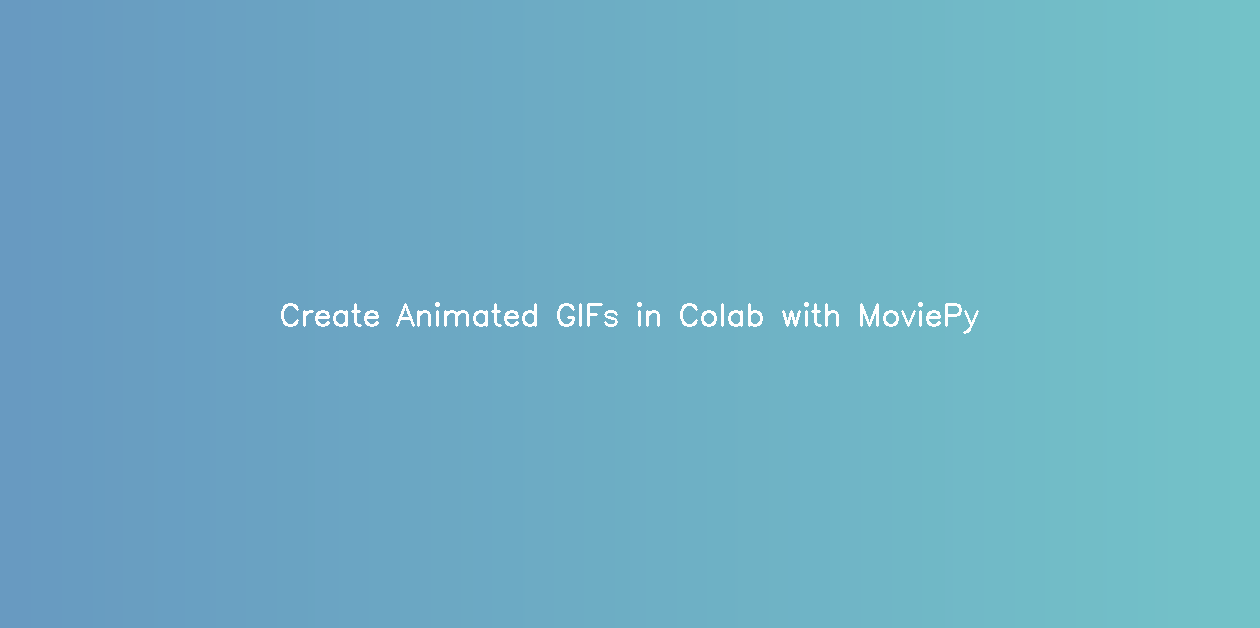
As of 2024, the day I am writing this article, media content is one of the popular formats. Creating GIFs with any app is simple stuff, anyone can install the app or use the online version select images, length, and size and hit the generate button. But, what if we can do this same thing programmatically?
See, we programmers are a different breed who sometimes love to make software that will automate a task that would have been completed within a fraction of a second. But, that’s what makes us different.
In this article, we will share an interesting piece of code, that will demonstrate, how to generate gif programmatically. You can repurpose this code to implement your version as per your needs. The interesting thing about this article is, that, we will program it on Google Colab.
For people who don’t know what Google Colab is. It is a web platform provided by Google that provides a readymade environment for coding your programs, AIML training and much more. Python is built into it and so many libraries are preinstalled, you need to import them as needed.
You can even install your favourite package (specific version) there. Just use !pip command.
So, without further ado, let’s get started. Before moving forward if you find this article resonating with your tribe, kindly consider it on social media and let the discussion begin.
Animated GIFs are a popular way to share short, looping animations to the web. With the rise of tools like Google Colab, creating animated GIFs has become more accessible than ever.
In this tutorial, we’ll explore how to generate animated GIFs using the MoviePy library in Google Colab, a cloud-based Python environment.
Prerequisites:
- Basic understanding of Python programming language.
- Familiarity with Google Colab environment.
- Basic knowledge of image processing concepts.
Step 1: Setup Environment
First, we need to set up our environment. If you’re not already familiar with Google Colab, you can access it through your browser and create a new notebook.
Step 2: Import Libraries
Next, we’ll import the necessary libraries. We’ll be using MoviePy for creating the animated GIF, NumPy for generating random frames, and IPython.display for displaying the GIF in the notebook.
from moviepy.editor import VideoClip
import numpy as np
from IPython.display import Image, display
Step 3: Define Frame Generation Function
We’ll define a function to generate frames for our GIF. In this example, we’ll generate random frames of a specified width and height.
width = 640
height = 480
def make_frame(t):
frame = np.random.randint(0, 256, (height, width, 3), dtype=np.uint8)
return frame
Step 4: Create Video Clip
Now, we’ll create a VideoClip object using MoviePy, specifying the duration of the clip in seconds.
clip = VideoClip(make_frame, duration=5) # Duration is 5 seconds
Step 5: Save as GIF
We’ll save the video clip as an animated GIF by calling the write_gif method and specifying the output path and frames per second (fps).
output_path = 'animated.gif'
clip.write_gif(output_path, fps=10)
Step 6: Display Preview
Finally, we’ll display a preview of the generated GIF in the notebook using IPython.display.
print(f"Animated GIF saved at {output_path}")
display(Image(filename=output_path))
In this tutorial, we’ve learned how to create animated GIFs in Google Colab using the MoviePy library. Following these simple steps, you can easily generate and share your custom animations right from your browser.
Full Code: Paste this in Colab and Run.
This code is generating frames programmatically, you need to repurpose this part for your images.
from moviepy.editor import VideoClip
import numpy as np
from google.colab import files
from IPython.display import Image, display
# Define the dimensions of the image
width = 640
height = 480
# Create a function to generate frames
def make_frame(t):
# Generate a random frame
frame = np.random.randint(0, 256, (height, width, 3), dtype=np.uint8)
return frame
# Create the video clip
clip = VideoClip(make_frame, duration=5) # Duration is 5 seconds
# Save the video clip as an animated GIF
output_path = 'animated.gif'
clip.write_gif(output_path, fps=10)
# Display success message
print(f"Animated GIF saved at {output_path}")
# Display the preview
display(Image(filename=output_path))